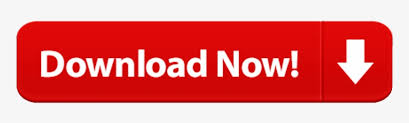
Insertion Operation -> In ArrayList inserting a new element at a specific position is costly O(n),as new space need to be created and each elements need to be moved by 1 position ahead.LinkedList has two pointers previous and next which points to before and after neighbouring elements,so insertion requires only adjustment of these pointers and insertion performance in LinkedList in O(1).Search Operation -> ArrayList search operation is faster compared to the LinkedList search operation.As ArrayList elements are index based, get(int index) in ArrayList gives the performance of O(1).But search operation performance in LinkedList is O(n) as elements need to be searched from the first node till the nth element node in average cases.Random access is possible in ArrayList using index.Random access is not possible in LinkedList.ArrayList supports binary search.LinkedList does not support binary search.Internal storage -> ArrayList internally uses dynamic array or resizable array to store the elements.LinkedList internally uses doubly linked list to store the elements.Both ArrayList and LinkedList implemnts List interface.But LinkedList implements Dequeue interface ,so LinkedList can be used as stack and also as Queue.Default capacity of ArrayList is 10 and default size of LinkedList is 0.You should use interface as type on the return type of method, type of arguments, etc. In fact, everywhere you need to declare a reference variable, you should always use the supertype, like Map for passing HashMap, Set for giving HashSet, and List for passing ArrayList, LinkedList, or Vector. This is known as programming for the interfaces.

Here we are using a List as a type of variable to store an object of ArrayList class, created using the new() operator. Why store the ArrayList object on the List variable? Always updated for the latest Java version like Java 12. This is the beginning, now let's see those two questions as well.Īnyway, If you are not familiar with Inheritance and other object-oriented programming concepts in Java, I suggest you first go through a comprehensive course like The Complete Java Masterclass, which is the most up-to-date online course to learn Java. This is known as Polymorphism because any virtual method will be executed from subclass only, even though they were called from the supertype. In Java or any object-oriented language, the supertype of a variable can store an object of subtype. Well, the main difference between List and ArrayList is that List is an interface while ArrayList is a class. Most importantly, it implements the List interface, which also means that ArrayList is a subtype of the List interface.
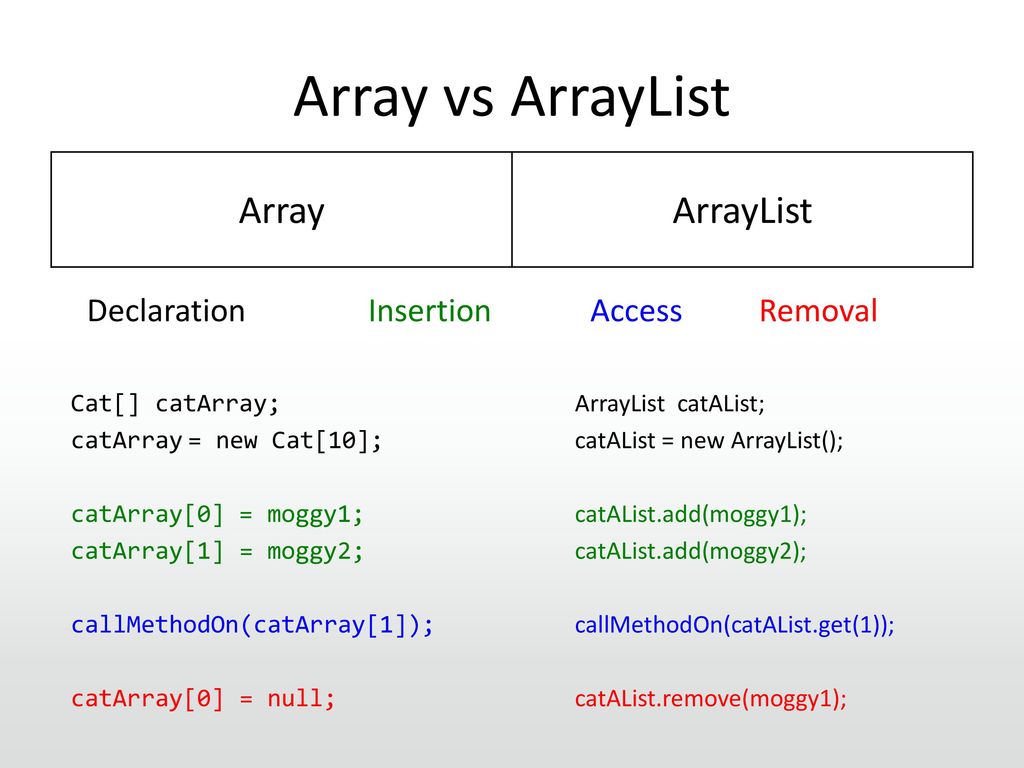
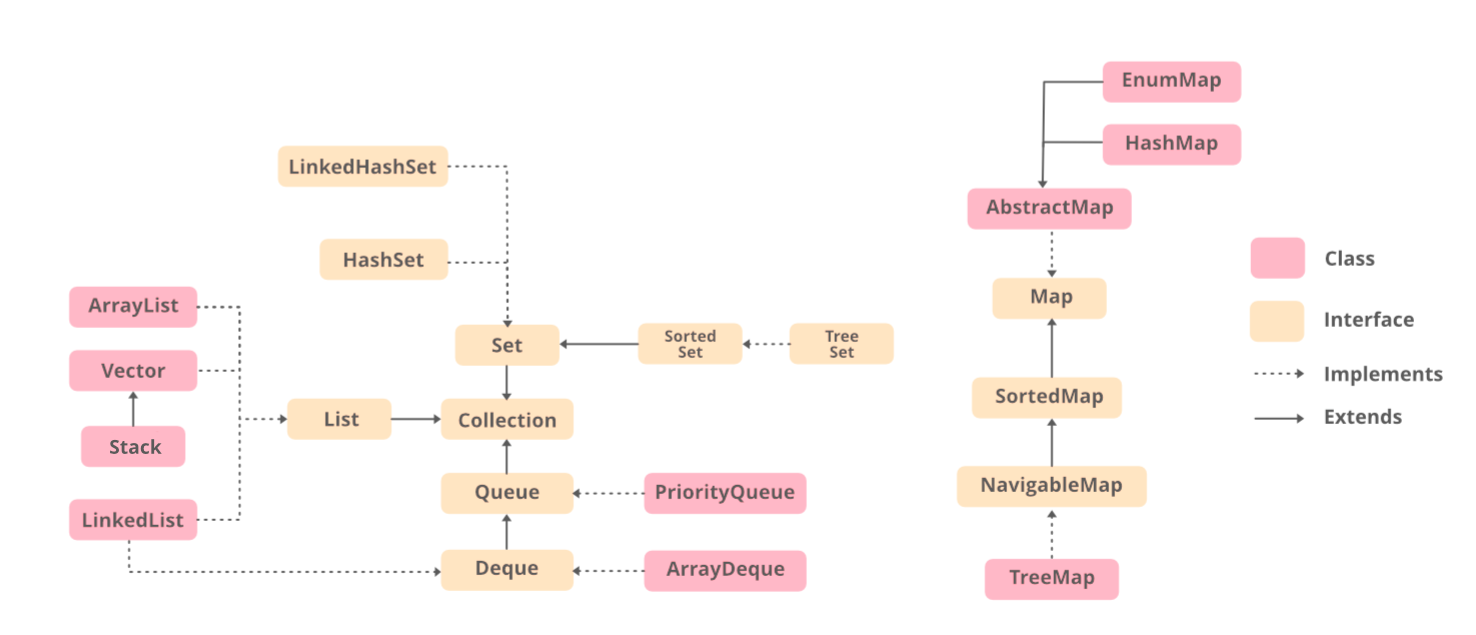

Someone who is just starting with Java programming language often has doubts about how we are storing an ArrayList object in List variable, what is the difference between List and ArrayList? Or why not just save the ArrayList object in the ArrayList variable just like we do for String, int, and other data types.
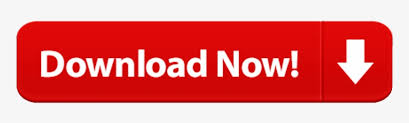